Last Updated On
The previous tutorial explained How to create Java Gradle project in Eclipse. In this tutorial, I will explain how we can set up a Gradle project with Selenium and TestNG.
Table of Contents
- Dependency List
- Implementation Steps
- Download and Install Java
- Download and setup Eclipse IDE on the system
- Setup Gradle
- Create a new Gradle Project
- Add Selenium and TestNG dependencies to the Gradle project
- Add Gradle Test Task to build.gradle
- Create Test Code under src/test/java
- Create testng.xml
- Run the tests from TestNG
- Run the tests from Command Line
- TestNG and Gradle Report generation
Dependency List
- Java 8 or above
- TestNG – 7.6.1
- Gradle – 7.5.1 (Build Tool)
- Selenium – 4.3.0
Implementation Steps
Step 1- Download and Install Java
Selenium needs Java to be installed on the system to run the tests. Click here to know How to install Java.
Step 2 – Download and setup Eclipse IDE on the system
The Eclipse IDE (integrated development environment) provides strong support for Java developers. Click here to know How to install Eclipse.
Step 3 – Setup Gradle
To build a test framework, we need to add several dependencies to the project. This can be achieved by any build tool. I have used Gradle Build Tool. Click here to know How to install Gradle.
Step 4 – Create a new Gradle Project
Below are the steps to create the Gradle project from command line.
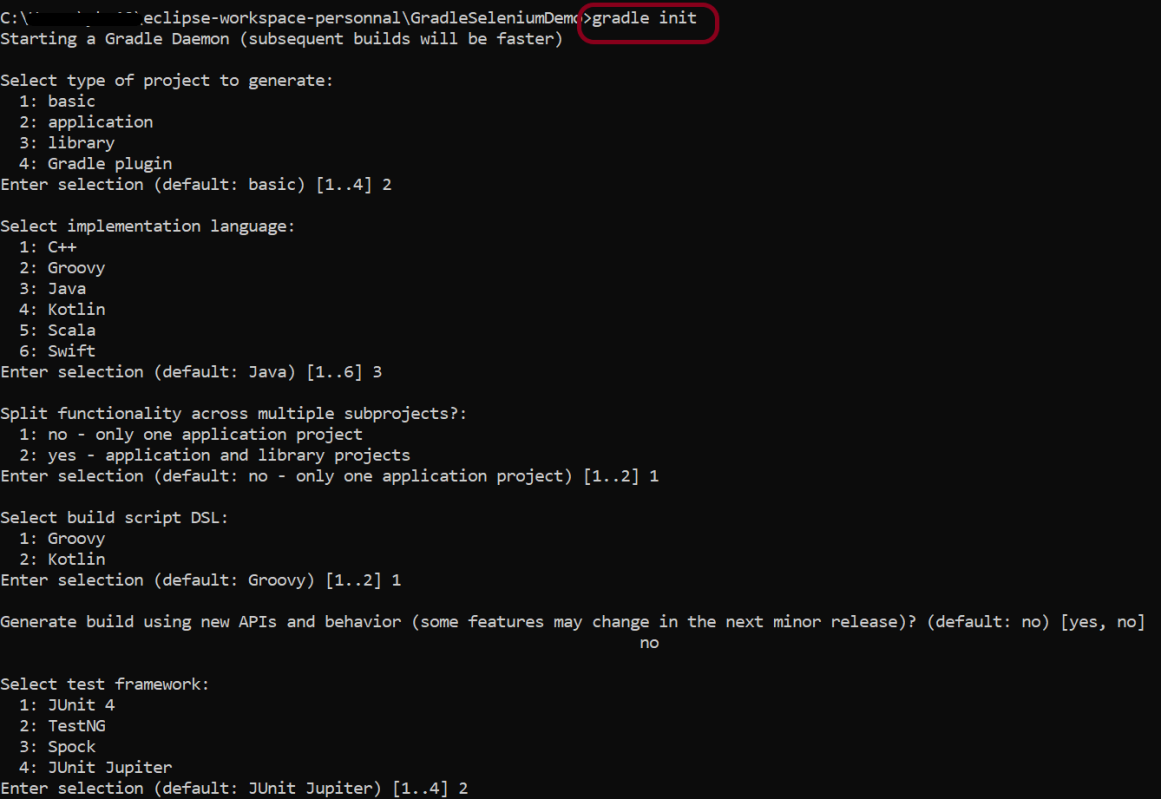

If you want to create the Gradle project from Eclipse IDE, click here to know How to create a Gradle Java project. Below is the structure of the Gradle project.

Step 5 – Add Selenium and TestNG dependencies to the Gradle project
dependencies {
// Use TestNG framework, also requires calling test.useTestNG() below
testImplementation 'org.testng:testng:7.4.0'
// This dependency is used by the application.
implementation 'com.google.guava:guava:31.0.1-jre'
implementation 'org.seleniumhq.selenium:selenium-java:4.4.0'
implementation 'io.github.bonigarcia:webdrivermanager:5.3.0'
}
Step 6 – Add Gradle Test Task to build.gradle
tasks.named('test') {
// Use TestNG for unit tests.
useTestNG() {
useDefaultListeners = true
outputDirectory = file("$projectDir/TestNG_Reports")
}
reports.html.setDestination(file("$projectDir/GradleReports"))
}
The complete gradle.build looks like something shown below.
*
* This file was generated by the Gradle 'init' task.
*
plugins {
// Apply the application plugin to add support for building a CLI application in Java.
id 'application'
}
repositories {
// Use Maven Central for resolving dependencies.
mavenCentral()
}
dependencies {
// Use TestNG framework, also requires calling test.useTestNG() below
testImplementation 'org.testng:testng:7.4.0'
// This dependency is used by the application.
implementation 'com.google.guava:guava:31.0.1-jre'
implementation 'org.seleniumhq.selenium:selenium-java:4.4.0'
implementation 'io.github.bonigarcia:webdrivermanager:5.3.0'
}
application {
// Define the main class for the application.
mainClass = 'com.example.App'
}
tasks.named('test') {
// Use TestNG for unit tests.
useTestNG() {
useDefaultListeners = true
outputDirectory = file("$projectDir/TestNG_Reports")
}
reports.html.setDestination(file("$projectDir/GradleReports"))
}
Step 7 – Create Test Code under src/test/java
Let us write the code to test a web application. I have created 3 tests and out of 3, 1 test will fail intentionally.
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.Assert;
import org.testng.annotations.AfterMethod;
import org.testng.annotations.BeforeMethod;
import org.testng.annotations.Test;
import io.github.bonigarcia.wdm.WebDriverManager;
public class LoginTests {
WebDriver driver;
@BeforeMethod
public void setUp() {
WebDriverManager.chromedriver().setup();
driver = new ChromeDriver();
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
driver.manage().window().maximize();
driver.get("https://opensource-demo.orangehrmlive.com/");
}
@Test(description = "This test validates error message when credentials are incorrect", priority = 0)
public void verifyIncorrectCredentials() {
driver.findElement(By.name("username")).sendKeys("Admin");
driver.findElement(By.name("password")).sendKeys("admin123$$");
driver.findElement(By.xpath("//*[@id='app']/div[1]/div/div[1]/div/div[2]/div[2]/form/div[3]/button")).submit();
String actualErrorMessage = driver.findElement(By.xpath("//*[@id='app']/div[1]/div/div[1]/div/div[2]/div[2]/div/div[1]/div[1]/p")).getText();
// Verify Error Message
Assert.assertEquals(actualErrorMessage,"Invalid credentials");
}
@Test(description = "This test will fail", priority = 1)
public void verifyBlankCredentials() {
driver.findElement(By.name("username")).sendKeys("");
driver.findElement(By.name("password")).sendKeys("admin123$$");
driver.findElement(By.xpath("//*[@id='app']/div[1]/div/div[1]/div/div[2]/div[2]/form/div[3]/button")).submit();
String actualErrorMessage = driver.findElement(By.xpath("//*[@id='app']/div[1]/div/div[1]/div/div[2]/div[2]/div/div[1]/div[1]/p")).getText();
// Verify Error Message
Assert.assertEquals(actualErrorMessage,"Invalid credentials");
}
@Test(description = "This test validates successful login to Home page", priority = 2)
public void verifyLoginPage() {
driver.findElement(By.name("username")).sendKeys("Admin");
driver.findElement(By.name("password")).sendKeys("admin123");
driver.findElement(By.xpath("//*[@id='app']/div[1]/div/div[1]/div/div[2]/div[2]/form/div[3]/button")).submit();
String homePageHeading = driver.findElement(By.xpath("//*[@id='app']/div[1]/div[2]/div[2]/div/div[1]/div[1]/div[1]/h5")).getText();
//Verify new page - HomePage
Assert.assertEquals(homePageHeading,"Employee Information");
}
@AfterMethod
public void teardown() {
driver.quit();
}
}
Step 8 – Create testng.xml
Right-click on the project and select TestNG and select Convert to TestNG.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE suite SYSTEM "https://testng.org/testng-1.0.dtd">
<suite name="Suite">
<test name="Selenium Tests with TestNG">
<classes>
<class name="com.example.LoginTests"/>
</classes>
</test> <!-- Test -->
</suite> <!-- Suite -->
Step 9 – Run the tests from TestNG
Right-Click on the testng.xml and select Run As TestNG Suite.
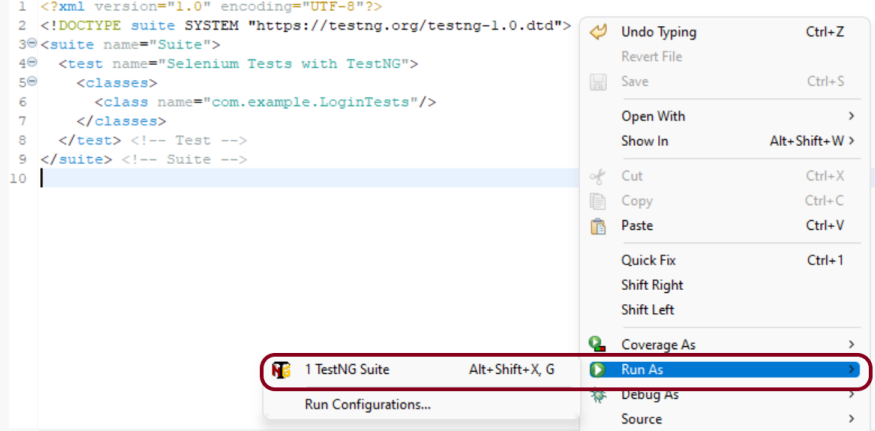
The output of the above tests in Eclipse Console is as shown below.

This also generates a folder with the name test-output that contains the TestNG reports like index.html, emailable-report.html.
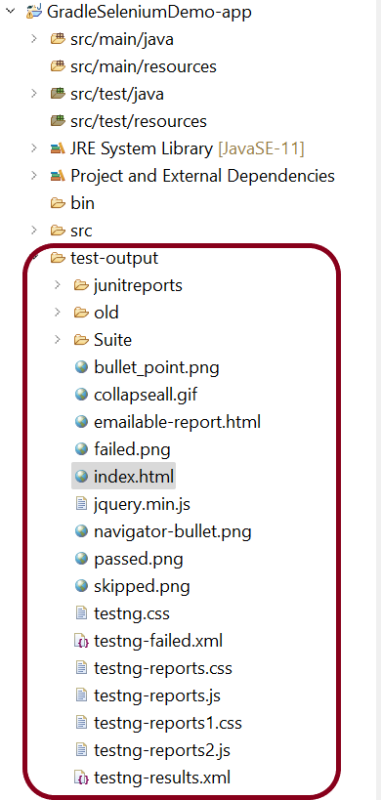
Step 10 – Run the tests from Command Line
To run the tests from the command line, use the below-mentioned command.
gradle clean test
The output of the above program is

Step 11 – TestNG and Gradle Report generation
Once the test execution is finished, refresh the project. We will see 2 folders – GradleReports and TestNG_ Reports.

Gradle Reports
This folder contains index.html.
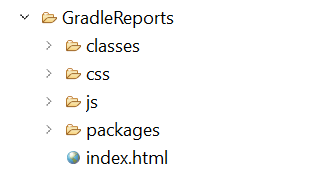
Right-click on index.html and select open with Web Browser. This report shows the summary of all the tests executed. As you can see that Failed tests are selected (highlighted in blue), so the name of the test failed along with the class name is displayed here.

TestNG Reports
Go to TestNG_Reports folder and right-click and open emailable-report.html.

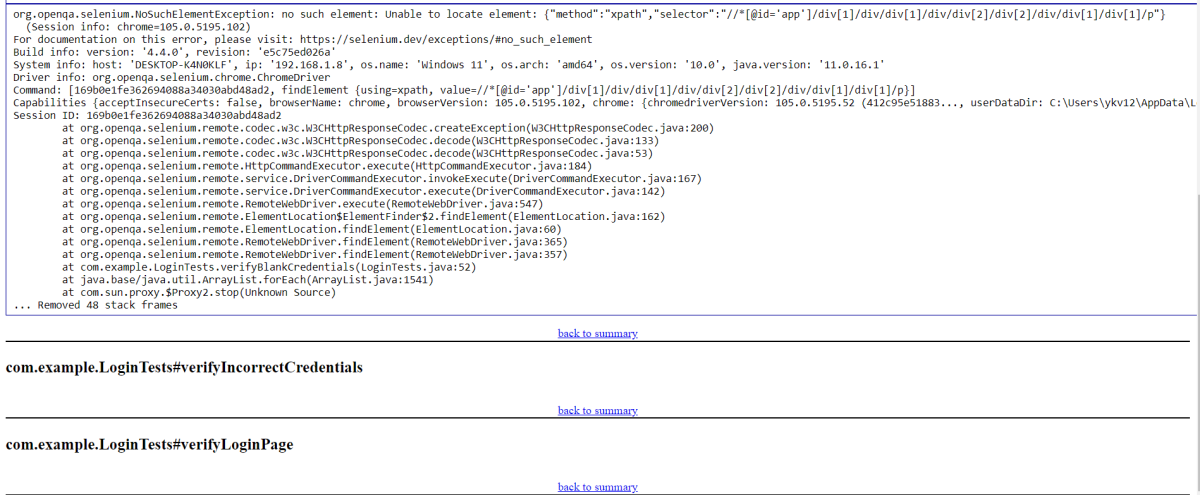
Index.html

Congratulations on making it through this tutorial and hope you found it useful! Happy Learning!! Cheers!!
Hi, unable to run using command prompt. i am not getting error just getting build successful message. why you have declared com.example.App in build.gradle file.
LikeLike
Hi Akash, you should be able to run the tests from command line using – gradle clean test. You can delete this part. This is auto-generated when the project is built as it contains an APP program.
LikeLike