Last Updated On
In this tutorial, we will see how can we retrieve the child nodes from an XML in Java.
What is XML?
XML stands for eXtensible Markup Language. It is a markup language much like HTML and was designed to store and transport data. XML was designed to be both human- and machine-readable. It is a flexible way to create information formats and share both the format and the data on the World Wide Web, intranets, and elsewhere.
Let’s see a simple XML.
<?xml version = "1.0"?>
<department>
<employee>
<firstname>Tom</firstname>
<lastname>Mathew</lastname>
<salary>25000</salary>
<age>21</age>
</employee>
</department>
1.A File object inputFile is created, representing the XML file located at “src/test/resources/testData/test.xml”.
2. A DocumentBuilderFactory instance is created. This is a factory API that enables applications to obtain a parser that produces DOM object trees from XML documents.
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
3. A DocumentBuilder instance is created from the factory. This API provides a way to parse XML documents and create a tree of Node objects.
DocumentBuilder dBuilder = dbFactory.newDocumentBuilder();
4. The XML document is parsed using the DocumentBuilder instance, resulting in a Document object that represents the entire XML document.
Document doc = dBuilder.parse(inputFile);
5. The normalize method is called on the Document object to ensure that the document’s DOM tree is fully normalized, which means that it is structurally correct according to the XML specification
doc.getDocumentElement().normalize();
6. It retrieves a NodeList of all employee elements in the document and prints out the number of such elements.
NodeList nodeList = doc.getElementsByTagName("employee");
System.out.println("Node Length :" + nodeList.getLength());
7. It iterates over each employee node in the NodeList. For each employee node, it prints out the node name and retrieves a NodeList of its child nodes.
for (int temp = 0; temp < nodeList.getLength(); temp++) {
Node node = nodeList.item(temp);
System.out.println("\nCurrent Element :" + node.getNodeName());
8. It then iterates over each child node of the employee node. If the child node is an element node (as opposed to other types of nodes like text nodes or comment nodes), it prints out the name and text content of the child node.
NodeList childNodes = node.getChildNodes();
for (int i = 0; i < childNodes.getLength(); i++) {
Node childNode = childNodes.item(i);
if (childNode.getNodeType() == Node.ELEMENT_NODE) {
System.out.println("Child node name " + i + ":" + childNode.getNodeName());
System.out.println("Child node value: " + i + ":" + childNode.getTextContent());
}
}
9. If any exceptions occur during the parsing process (such as ParserConfigurationException, SAXException, or IOException), they are caught, and their stack traces are printed out.
The complete program is mentioned below:
package com.example.XML;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import java.io.File;
import java.io.IOException;
public class ChildNodes {
public static void main(String[] args) {
try {
//Create a DocumentBuilder
File inputFile = new File("src/test/resources/testData/test1.xml");
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder = dbFactory.newDocumentBuilder();
Document doc = dBuilder.parse(inputFile);
doc.getDocumentElement().normalize();
//Extract the root element
System.out.println("Root element :" + doc.getDocumentElement().getNodeName());
NodeList nodeList = doc.getElementsByTagName("employee");
System.out.println("Node Length :" + nodeList.getLength());
for (int temp = 0; temp < nodeList.getLength(); temp++) {
Node node = nodeList.item(temp);
System.out.println("\nCurrent Element :" + node.getNodeName());
NodeList childNodes = node.getChildNodes();
for (int i = 0; i < childNodes.getLength(); i++) {
Node childNode = childNodes.item(i);
if (childNode.getNodeType() == Node.ELEMENT_NODE) {
System.out.println("Child node name " + i + ":" + childNode.getNodeName());
System.out.println("Child node value: " + i + ":" + childNode.getTextContent());
}
}
}
} catch (ParserConfigurationException | SAXException | IOException e) {
e.printStackTrace();
}
}
}
The output of the above program is

Complex XML
<?xml version = "1.0"?>
<department>
<employee>
<firstname>Tom</firstname>
<lastname>Mathew</lastname>
<salary>25000</salary>
<age>21</age>
</employee>
<employee id = "429">
<firstname>Erika</firstname>
<lastname>David</lastname>
<salary>
<fixed>
<fixed1>350000</fixed1>
<fixed2>200000</fixed2>
</fixed>
<bonus>5000</bonus>
</salary>
<age>29</age>
<type>Permanent</type>
</employee>
</department>
Let us create a program to get the child node of the above program:
package com.example.XML;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import java.io.File;
import java.io.IOException;
public class ComplexChildNodes {
public static void main(String[] args) {
try {
//Create a DocumentBuilder
File inputFile = new File("src/test/resources/testData/test2.xml");
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder = dbFactory.newDocumentBuilder();
Document doc = dBuilder.parse(inputFile);
doc.getDocumentElement().normalize();
//Extract the root element
System.out.println("Root element :" + doc.getDocumentElement().getNodeName());
NodeList nodeList = doc.getElementsByTagName("employee");
System.out.println("Node Length :" + nodeList.getLength());
for (int temp = 0; temp < nodeList.getLength(); temp++) {
Node node = nodeList.item(temp);
System.out.println("\nCurrent Element :" + node.getNodeName());
NodeList childNodes = node.getChildNodes();
for (int i = 0; i < childNodes.getLength(); i++) {
Node childNode = childNodes.item(i);
if (childNode.getNodeType() == Node.ELEMENT_NODE) {
System.out.println("Child node name " + i + ":" + childNode.getNodeName());
System.out.println("Child node value: " + i + ":" + childNode.getTextContent());
for (int j = 0; j < childNode.getChildNodes().getLength(); j++) {
Node subChildNode = childNode.getChildNodes().item(j);
if (subChildNode.getNodeType() == Node.ELEMENT_NODE) {
{
System.out.println("Sub Child node name " + j + ":" + subChildNode.getNodeName());
System.out.println("Sub Child node value: " + j + ":" + subChildNode.getTextContent());
}
}
}
}
}
}
} catch (ParserConfigurationException | SAXException | IOException e) {
e.printStackTrace();
}
}
}
The output of the above program is

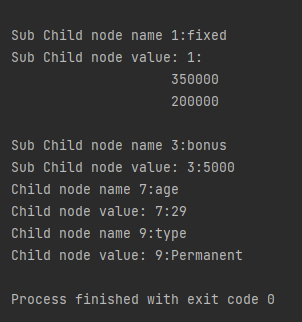