In JUnit5, JUnit Jupiter provides the ability to repeat a test a specified number of times by annotating a method with @RepeatedTest. We can specify the repetition frequency as a parameter to the @RepeatedTest annotation.
When do we use the Repeated Test?
Imagine, we are testing an online shopping website. In the process of placing an order, we need to pay for the product. But the payment gateway is a third-party service, and we cannot control the connectivity between our website and the payment gateway. We know that clicking on the ‘Payment’ link sometime shows “Exception: Page cannot be displayed”. This is an intermittent issue. So, we don’t want to fail the test if this payment link does not work. We can configure it to click this payment link multiple times, before marking the test case failed. Here comes the Repeated Test in the picture.
A few points to keep in mind for @RepeatedTest are as follows:
- The methods annotated with @RepeatedTest cannot be static, otherwise, the test cannot be found.
- The methods annotated with @RepeatedTest cannot be private, otherwise, the test cannot be found.
- The return type of the method annotated with @RepeatedTest must be void only, otherwise, the test cannot be found.
The below example will run the test 5 times.
@DisplayName("Addition")
@RepeatedTest(3)
void repeatTest(){
int a = 4;
int b = 6;
assertEquals(10, a+b,"Incorrect sum of numbers");
}
The output of the above test:
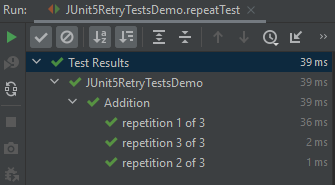
Each invocation of a repeated test behaves like the execution of a regular @Test
method, with full support for the same lifecycle callbacks and extensions.
It means that @BeforeEach and @AfterEach annotated lifecycle methods will be invoked for each invocation of the test.
@BeforeEach annotation is used to signal that the annotated method should be executed before each invocation of the @Test, @RepeatedTest, @ParameterizedTest, or @TestFactory method in the current class. This is the replacement of the @Before Method in JUnit4.
TestInfo is used to inject information about the current test or container into @Test, @RepeatedTest, @ParameterizedTest, @TestFactory, @BeforeEach, @AfterEach, @BeforeAll, and @AfterAll methods.
If a method parameter is of type TestInfo, JUnit will supply an instance of TestInfo corresponding to the current test or container as the value for the parameter.
RepetitionInfo is used to inject information about the current repetition of a repeated test into the @RepeatedTest, @BeforeEach, and @AfterEach methods.
If a method parameter is of type RepetitionInfo, JUnit will supply an instance of RepetitionInfo corresponding to the current repeated test as the value for the parameter.
In the below example, @BeforeEach will get executed before each repetition of each repeated test. By having the TestInfo and RepetitionInfo injected into the method, we see that it’s possible to obtain information about the currently executing repeated test.
import org.junit.jupiter.api.*;
import static org.junit.jupiter.api.Assertions.*;
public class RepeatCycleDemo {
@BeforeEach
void init(TestInfo testInfo, RepetitionInfo repetitionInfo) {
System.out.println("Before Each init() method called");
int currentRepetition = repetitionInfo.getCurrentRepetition();
int totalRepetitions = repetitionInfo.getTotalRepetitions();
String methodName = testInfo.getTestMethod().get().getName();
System.out.println(String.format("About to execute repetition %d of %d for %s", currentRepetition, totalRepetitions, methodName));
}
@RepeatedTest(3)
void repeatedTestWithRepetitionInfo(RepetitionInfo repetitionInfo) {
int a = 4;
int b = 6;
assertEquals(10, a+b, repetitionInfo.getTotalRepetitions());
}
@AfterEach
public void cleanUpEach(){
System.out.println("=================After Each cleanUpEach() method called =================");
}
}
The output of the above test:
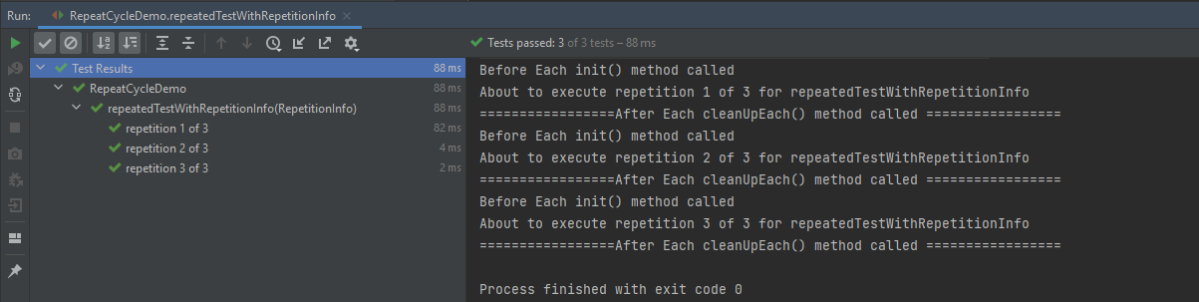
Custom Display Name
In addition to specifying the number of repetitions, a custom display name can be configured for each repetition via the name attribute of the @RepeatedTest annotation.
The display name can be a pattern composed of a combination of static text and dynamic placeholders. The following placeholders are currently supported.
- {displayName}: display name of the @RepeatedTest method
- {currentRepetition}: the current repetition count
- {totalRepetitions}: the total number of repetitions
@BeforeEach
void init(TestInfo testInfo, RepetitionInfo repetitionInfo) {
System.out.println("Before Each init() method called");
int currentRepetition = repetitionInfo.getCurrentRepetition();
int totalRepetitions = repetitionInfo.getTotalRepetitions();
String methodName = testInfo.getTestMethod().get().getName();
System.out.println(String.format("About to execute repetition %d of %d for %s", //
currentRepetition, totalRepetitions, methodName));
}
//Custom Display
@RepeatedTest(value = 2, name = "{displayName} {currentRepetition}/{totalRepetitions}")
@DisplayName("Repeat JUnit5 Test")
void customDisplayName(TestInfo testInfo) {
assertEquals("Repeat JUnit5 Test 1/2", testInfo.getDisplayName());
}
The output of the above test:
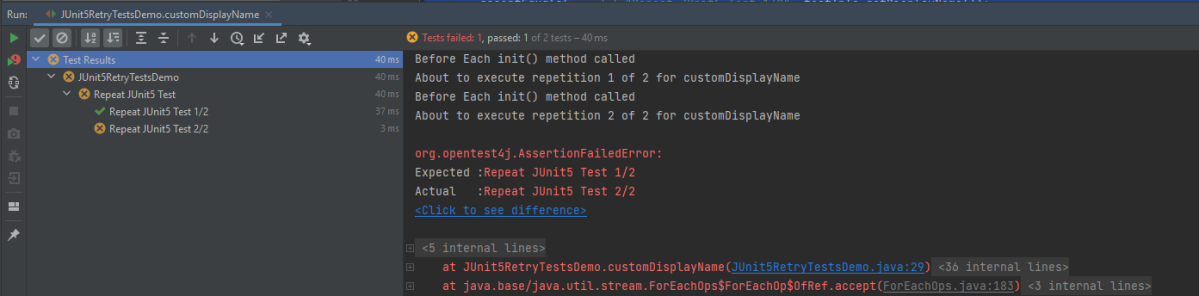
The default display name for a given repetition is generated based on the following pattern: “repetition {currentRepetition} of {totalRepetitions}”. Thus, the display names for individual repetitions of the previous repeatedTest() example would be repetition 1 of 10, and repetition 2 of 10.
We can use one of two predefined formats for displaying the name – LONG_DISPLAY_NAME and SHORT_DISPLAY_NAME. The latter is the default format if none is specified.
- RepeatedTest.LONG_DISPLAY_NAME – {displayName} :: repetition {currentRepetition} of {totalRepetitions}
- RepeatedTest.SHORT_DISPLAY_NAME – repetition {currentRepetition} of {totalRepetitions}
Below is an example of SHORT_DISPLAY_NAME
@RepeatedTest(value = 3, name = RepeatedTest.SHORT_DISPLAY_NAME)
@DisplayName("Multiplication")
void customDisplayNameWithShortPattern() {
assertEquals(8, 6*5);
}
In this case, the name is displayed as Multiplication repetition 1 of 3, repetition 2 of 3, and soon.
The output of the above test:

Below is an example of LONG_DISPLAY_NAME
@RepeatedTest(value = 3, name = RepeatedTest.LONG_DISPLAY_NAME)
@DisplayName("Addition")
void customDisplayNameWithLongPattern(TestInfo testInfo) {
System.out.println("Display Name :"+ testInfo.getDisplayName());
System.out.println("Test Class Name :"+ testInfo.getTestClass());
System.out.println("Test Method :"+ testInfo.getTestMethod());
assertEquals(8, 3+7);
}
The output of the above test:
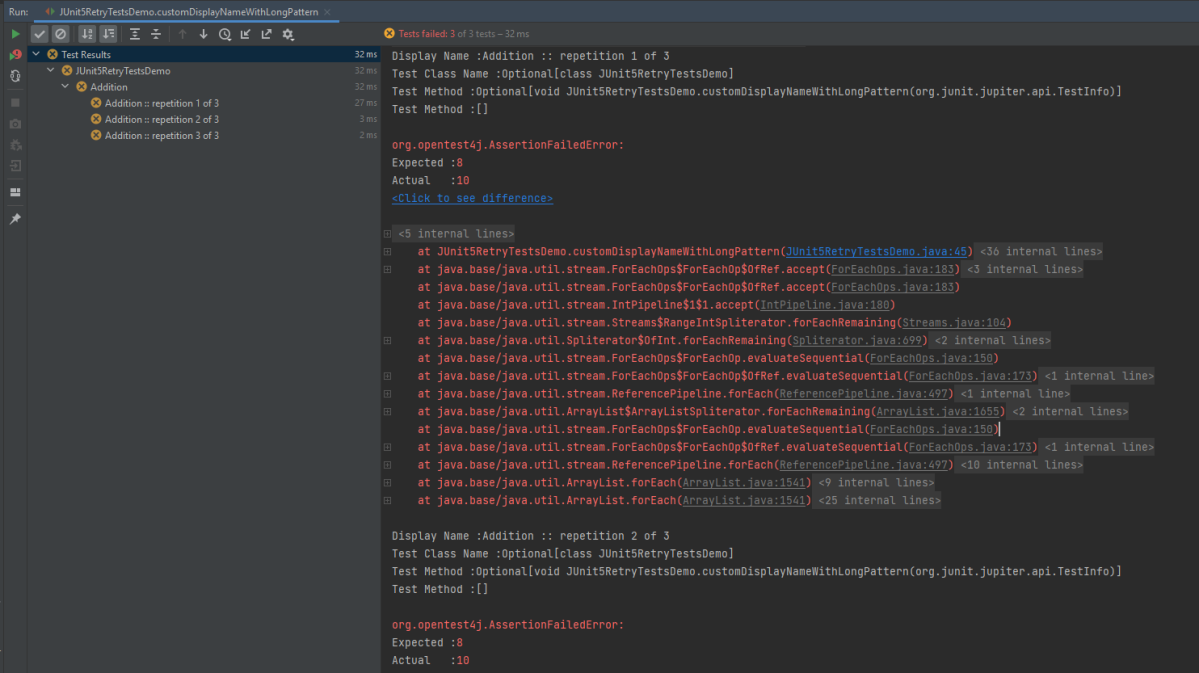
As we can see in the example, we have used @DisplayName(“Addition”), but as it is name = RepeatedTest.LONG_DISPLAY_NAME, so the name of the tests are now Addition :: repetition 1 of 3, Addition :: repetition 2 of 3 and soon.
Congratulations. We are able to execute the tests multiple times by using the @RepeatedTest annotation. Happy Learning!!