In this tutorial, we’ll explore how to carry out Cross-Browser testing using PyTest.
Table of Contents
- What is Cross-Browser Testing?
- Why do we need to perform Cross Browser Testing?
- Prerequisite
- Implementation Steps
What is Cross-Browser Testing?
Cross Browser is a technique in which a web application tests on different browsers and operating systems. Cross Browser testing, make sure that the site is rendered the same in every browser and operating system, and ensure that the users have a good experience.
Why do we need to perform Cross Browser Testing?
User Experience Consistency: Different browsers can render web content differently. Cross-browser testing ensures that users have a consistent and reliable experience regardless of the browser they choose.
Browser-Specific Issues: Each browser has its quirks, bugs, and features. Different browser is compatible with different operating systems. Cross-browser testing helps identify and address browser-specific issues or inconsistencies that may affect functionality or appearance.
Prerequisite:
- Python is installed on the machine
- PIP is installed on the machine
- PyCharm or another Python Editor is installed on the machine
If you need help in installing Python, please refer to this tutorial – How to Install Python on Windows 11.
If you need help in installing PyCharm, please refer to this tutorial – How to install PyCharms on Windows 11.
Implementation Steps
1. Parameterize the test in conftest.py
PyTest allows easy parameterization of tests. To run a test against a list of browsers, we can pass the browser_name through the command line.
import pytest
from selenium import webdriver
def pytest_addoption(parser):
"""Custom Pytest command line options"""
parser.addoption(
"--browser_name", action="store", default="chrome"
)
@pytest.fixture(scope="class")
def driver(request):
browser_name = request.config.getoption("browser_name")
if browser_name == "chrome":
options = webdriver.ChromeOptions()
driver = webdriver.Chrome(options)
print("Chrome Browser is opened")
elif browser_name == "firefox":
options = webdriver.FirefoxOptions()
driver = webdriver.Firefox(options)
print("Firefox Browser is opened")
elif browser_name == "edge":
options = webdriver.EdgeOptions()
driver = webdriver.Edge(options)
print("Edge Browser is opened")
else:
print("No browser is selected")
driver.get("https://opensource-demo.orangehrmlive.com/")
driver.maximize_window()
driver.implicitly_wait(5)
yield driver
driver.close()
If we do not pass any browser_name through the command line, then the test will run by default using Chrome.
2. Create the tests
Below is an example of the test.
from selenium.webdriver.common.by import By
class Test_Login:
def test_login(self,driver):
driver.find_element(By.NAME, "username").send_keys("Admin")
driver.find_element(By.NAME, "password").send_keys("admin123")
driver.find_element(By.XPATH, "//*[@class='oxd-form']/div[3]/button").click()
print("Successful login to the application")
homePageTitle = driver.find_element(By.XPATH, "//*[@class='oxd-topbar-header-breadcrumb']/h6").text
print("Heading of DashBoard Page: ", homePageTitle)
assert homePageTitle == "Dashboard", "Heading is different"
3. Execute the tests
To run the tests through the command line, use the below command:
pytest test_login.py -s
This will execute the tests on the Chrome browser, as we have not passed any browser_name through the command line.
The output of the above program is
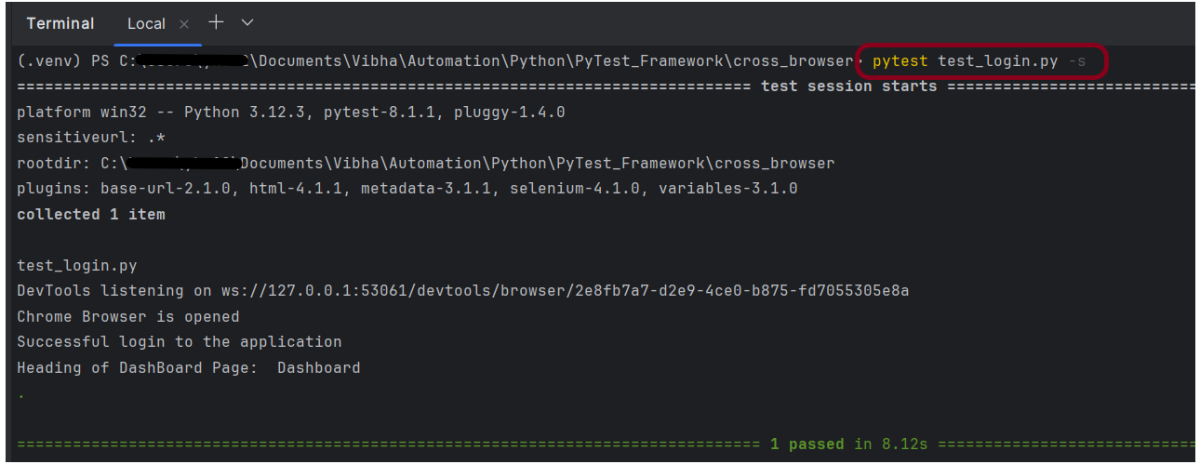
If we want to run the tests on the firefox browser, then use the below command:
pytest test_login.py --browser_name firefox -s
The output of the above program is
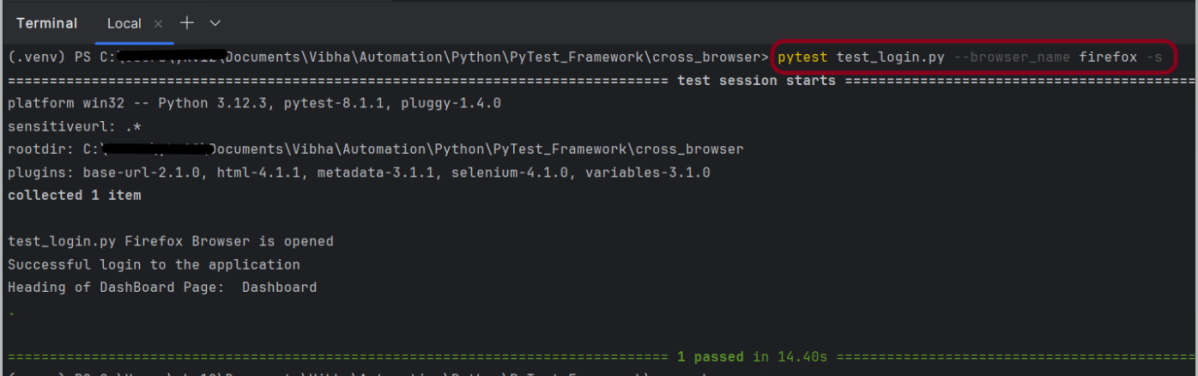
Similarly, we can execute the tests in the edge browser by just changing the browser_name in the command line.
Congratulations on making it through this tutorial and hope you found it useful! Happy Learning!! Cheers!!