In this tutorial, I will explain the conversion of JSON Object (payload) to JAVA Object. We will use Gson API for the same purpose.
Before going through this tutorial, spend some time understanding Serialization using Gson API.
We can parse the JSON or XML response into POJO classes. After parsing into POJO classes, we can easily get values from the response easily. This is called De-serialization. For this, we can use any JSON parser APIs. Here, we are going to use Gson API.
To start with, add the below dependency to the project.
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.9</version>
</dependency>
Sample JSON Payload
{
"firstName" : "Vibha",
"lastName" : "Singh",
"age" : 30,
"salary" : 75000.0,
"designation" : "Manager",
"contactNumber" : "+91999996712",
"emailId" : "abc123@test.com"
}
Let us create a class called Employee with a field name exactly (case-sensitive) the same as node names in the above JSON string because with the default setting while parsing JSON object to Java object, it will look on getter setter methods of field names.
public class Employee {
// private variables or data members of POJO class
private String firstName;
private String lastName;
private int age;
private double salary;
private String designation;
private String contactNumber;
private String emailId;
// Getter and setter methods
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
public String getDesignation() {
return designation;
}
public void setDesignation(String designation) {
this.designation = designation;
}
public String getContactNumber() {
return contactNumber;
}
public void setContactNumber(String contactNumber) {
this.contactNumber = contactNumber;
}
public String getEmailId() {
return emailId;
}
public void setEmailId(String emailId) {
this.emailId = emailId;
}
}
Gson class provides multiple overloaded fromJson() methods to achieve this. Below is a list of available methods:-
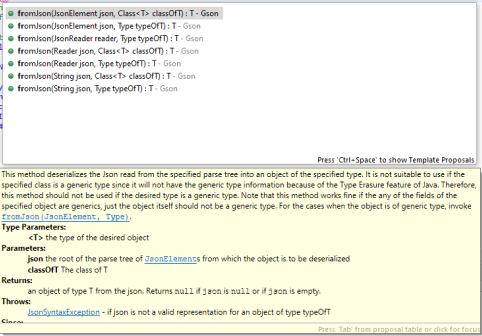
In the below test, I have mentioned the JSON Payload string in the test and used Gson API to deserialize the JSON payload to JAVA Object.
@Test
public void getDetailFromJson() {
// De-serializing from JSON String
String jsonString = "{\r\n" + " \"firstName\": \"Tom\",\r\n" + " \"lastName\": \"John\",\r\n"
+ " \"age\": 30,\r\n" + " \"salary\": 50000.0,\r\n" + " \"designation\": \"Lead\",\r\n"
+ " \"contactNumber\": \"+917642218922\",\r\n" + " \"emailId\": \"abc@test.com\"\r\n" + "}";
Gson gson = new Gson();
// Pass JSON string and the POJO class
Employee employee = gson.fromJson(jsonString, Employee.class);
// Now use getter method to retrieve values
System.out.println("Details of Employee is as below:-");
System.out.println("First Name : " + employee.getFirstName());
System.out.println("Last Name : " + employee.getLastName());
System.out.println("Age : " + employee.getAge());
System.out.println("Salary : " + employee.getSalary());
System.out.println("designation : " + employee.getDesignation());
System.out.println("contactNumber : " + employee.getContactNumber());
System.out.println("emailId : " + employee.getEmailId());
System.out.println("########################################################");
}
The output of the above program is

We can get the JSON payload from a file present in a project under src/test/resources as shown in the below image.


public class EmployeeDeserializationGsonTest {
@Test
public void fromFile() throws FileNotFoundException {
Gson gson = new Gson();
// De-serializing from a json file
String userDir = System.getProperty("user.dir");
File inputJsonFile = new File(userDir + "\\src\\test\\resources\\EmployeePayloadUsingGson.json");
FileReader fileReader = new FileReader(inputJsonFile);
Employee employee1 = gson.fromJson(fileReader, Employee.class);
// Now use getter method to retrieve values
System.out.println("Details of Employee is as below:-");
System.out.println("First Name : " + employee1.getFirstName());
System.out.println("Last Name : " + employee1.getLastName());
System.out.println("Age : " + employee1.getAge());
System.out.println("Salary : " + employee1.getSalary());
System.out.println("designation : " + employee1.getDesignation());
System.out.println("contactNumber : " + employee1.getContactNumber());
System.out.println("emailId : " + employee1.getEmailId());
System.out.println("########################################################");
}
}
The output of the above program is
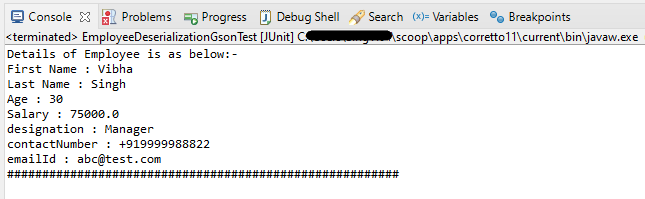
We are done! Congratulations on making it through this tutorial and hope you found it useful! Happy Learning!!